Building a High-Performance Multi-Threaded Audio Processing System
Core principles, strategies, and techniques for designing an efficient multi-threaded audio processing system.
Creating a high-performance multi-threaded audio processing system requires meticulous attention to multiple factors, especially when dealing with real-time audio. Real-time audio processing has strict performance and latency requirements, where any inefficiency or delay in the audio thread can lead to glitches, dropouts, or buffer underruns. Below are the core principles, strategies, and techniques for designing an efficient multi-threaded audio processing system.
Understanding Real-Time Constraints
In real-time audio processing, the system must process audio within a fixed time frame, determined by the buffer size and sample rate. For instance, with a buffer size of 512 samples and a sample rate of 44.1 kHz, the audio callback must process these samples within a time window of:
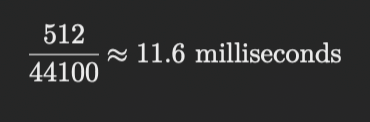
Failure to complete processing within this time frame causes an audio buffer underflow, leading to glitches or dropouts.
Key Concepts for Multi-Threaded Audio Processing
When introducing multi-threading into audio processing, several essential concepts and practices are critical:
• Thread Safety: Carefully manage shared resources to prevent race conditions and contention.
• Lock-Free Design: Whenever possible, use lock-free structures to avoid blocking the real-time audio thread
.• Work Distribution: Efficiently distribute processing tasks across threads.
Thread Safety
Thread safety ensures that shared data or resources are accessed consistently and correctly when multiple threads are running concurrently. It’s crucial to avoid issues like data races, deadlocks, and other unpredictable behaviors in multi-threaded systems. Thread safety is typically achieved through synchronization mechanisms such as mutexes, condition variables, semaphores, and atomic operations.
Best practices for thread safety include:
1. Minimize Shared Data: Reducing the amount of shared data minimizes the need for synchronization.
2. Limit Lock Scope: Hold locks for the shortest time necessary to reduce contention and improve performance.
3. Choose the Right Synchronization Mechanism: Use atomic operations for simple updates, shared mutexes for read-heavy data, and condition variables for signaling.
4. Maintain Consistent Lock Order: Always acquire locks in a consistent order across threads to avoid deadlocks.
5. Use RAII for Lock Management: C++’s std::lock_guard and std::unique_lock automatically manage locks, ensuring they are released even if exceptions occur.
Lock-Free Design
Never block the real-time audio thread. Any delay in the audio thread can lead to audible glitches or noise.To minimize synchronization needs in your audio code, rely on std::atomics, lock-free FIFOs, immutable data structures, and keep processing code separate from the data model whenever possible. (If a lock is absolutely necessary in the audio thread, refer to this resource for best practices.)
A ring buffer (or circular buffer) is a widely used data structure in audio applications that enables lock-free communication between two threads. A ring buffer enables efficient, non-blocking data sharing using two pointers or indexes: one for writing (writeIndex) and one for reading (readIndex). For a well-implemented lock-free queue, refer to this repository.
Work Distribution
Efficient work distribution is a key benefit of multi-threading in audio processing, as it maximizes CPU utilization by keeping all threads active. In a typical audio processing system, raw audio undergoes multiple processing steps and mixing before reaching the audio output. An audio graph is often used to organize this complex processing flow. An audio project may contain multiple tracks, with each track holding various nodes such as effects, audio sources, mixers, etc. These nodes interconnect to form an audio graph, through which data flows between nodes. Treating nodes as the smallest unit of processing enables the highest level of parallelism. For a deeper understanding of audio graphs, check out this talk.
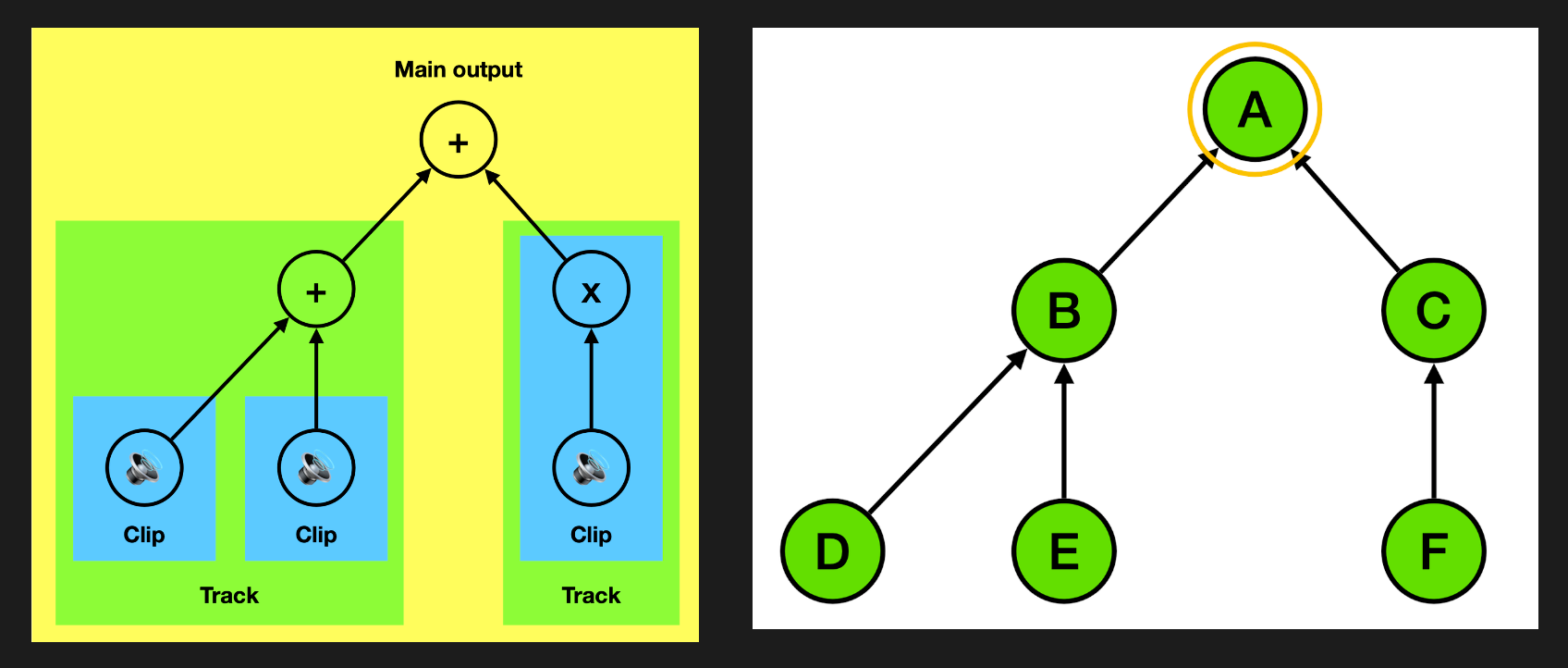
Node Execution Order
Since shallow nodes depend on data from deeper nodes, we must process deeper nodes first. By representing the graph as an N-ary tree, we can use post-order Depth-First Search (DFS) to determine an execution order.
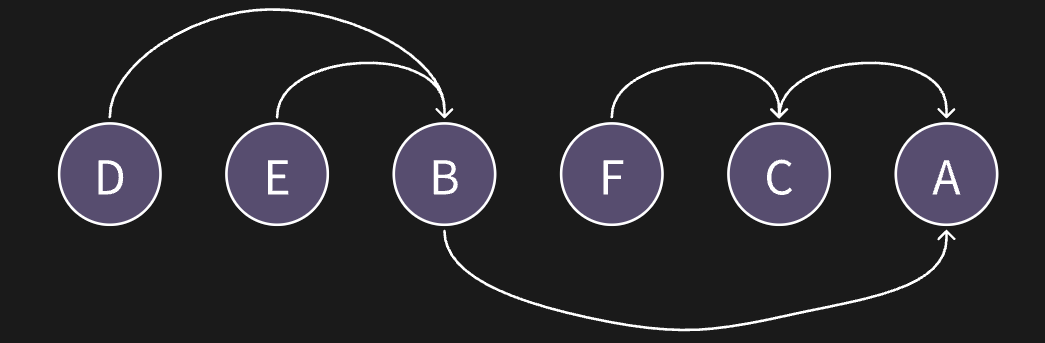
Simply defining an execution order isn’t enough for parallel processing; it’s also essential to determine each node’s dependencies. Once we have all the dependency information, we can distribute tasks across worker threads and task queues. Here’s an example according to the diagram above:
1. The audio thread generates audio tasks: ABCDEF
2. Tasks are enqueued in a lock-free queue or circular buffer.
3. Worker threads dequeue and process tasks in parallel:
- Tasks D, E, and F have no dependencies, so these can be processed concurrently first.
- Once F completes, C becomes available and can start processing.
- Once D and E complete, B can be processed.
- Finally, A can be processed to fill the final audio buffer.
4. The audio thread periodically checks the results and assembles the final audio buffer.

Another important consideration in work distribution is Delay Compensation. In an audio graph, delay compensation ensures that all audio signals arrive at the output (or the next processing stage) in sync, even if different nodes introduce varying amounts of processing delay. This is essential in real-time audio systems, where delays between different audio paths can cause phase issues, timing inconsistencies, and a loss of stereo image.
How Delay Compensation Works
Delay compensation involves calculating the delay for each node and adding “compensatory delays” where needed to ensure signals align at each critical point in the graph. Here’s how it typically works:
1. Calculate the Delay of Each Node: Each node in the audio graph specifies its processing delay, either as a fixed value or dynamically based on its configuration. The system propagates these delays through the audio graph, summing the delays for each signal path to calculate the total delay for each path.
2. Determine the Maximum Path Delay: Identify the longest delay path, as this will dictate the necessary delay compensation for shorter paths. The audio engine uses this maximum delay as the reference delay.
3. Add Compensatory Delays to Shorter Paths: For paths with less delay than the maximum path, add compensatory delay nodes (or “dummy delays”) to equalize delay across all paths. This ensures that all signals, regardless of their path, reach the output in sync.
4. Adjust for Real-Time Processing: In real-time processing, delay compensation should be limited to what the system latency allows. Excessive compensatory delay may increase latency, affecting real-time performance. Real-time systems balance delay compensation to reduce timing issues without excessive latency.
Example of Delay Compensation in an Audio Graph
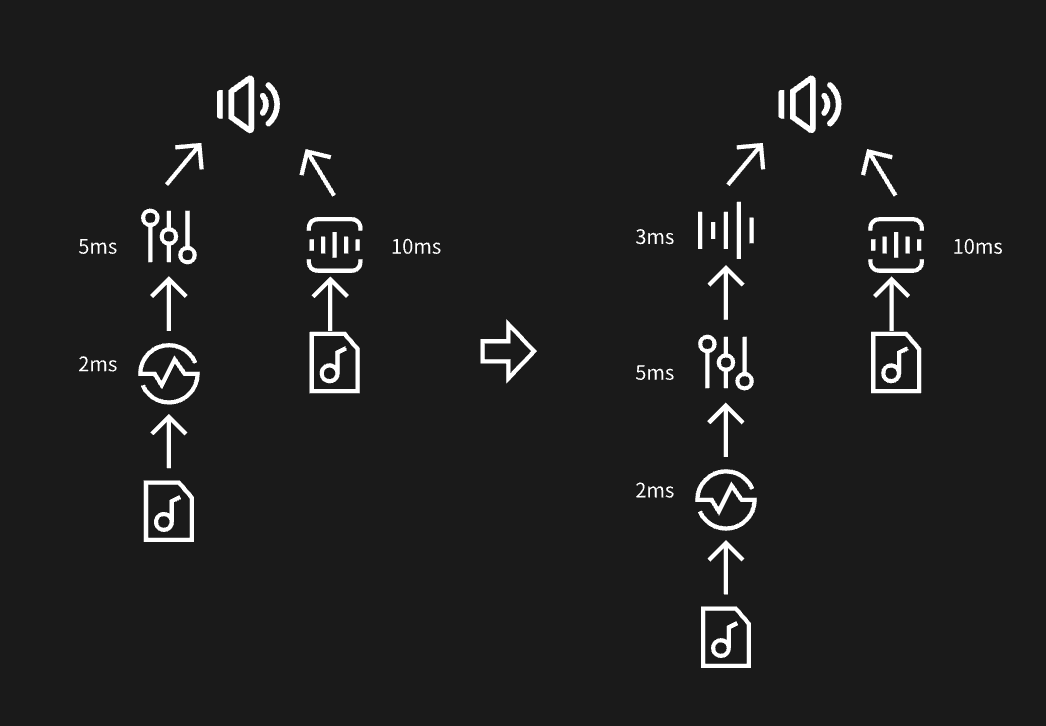
Consider an audio graph with nodes in two separate paths:
• Path A: Input → Equalizer (2ms delay) → Compressor (5ms delay) → Output
• Path B: Input → Reverb (10ms delay) → Output
Steps:
- Calculate Path Delays:• Path A has a total delay of 2ms + 5ms = 7ms.• Path B has a total delay of 10ms.
- Determine the Maximum Delay:• Path B has the maximum delay of 10ms.
- Add Compensatory Delays:• To equalize delays, add a 3ms compensatory delay to Path A.Now, both paths have an equal 10ms delay, ensuring synchronized audio at the output.
Task Allocation Across Audio Callbacks. By distributing audio tasks among different threads, each callback in the audio thread can leverage concurrent processing. However, if the number of threads exceeds the available tasks per callback, some threads may remain idle. To address this, consider adding a lock-free FIFO buffer and an additional producer-consumer thread to pre-process multiple audio frames, increasing parallel bandwidth.
Thread Priority Matters. To achieve high performance in real-time audio processing, thread priority settings are essential. Assigning a high priority (or real-time priority, if supported) to the audio thread ensures it receives immediate CPU time when needed.
Performance Optimization Techniques
- Minimize Context Switching: Efficiently distribute workloads and use techniques like thread affinity to reduce context-switching overhead.
- SIMD (Single Instruction, Multiple Data): For DSP-intensive tasks, leverage SIMD instructions (e.g., AVX, SSE) to process multiple audio samples simultaneously.
- Efficient Memory Management: Pre-allocate memory and reuse buffers to avoid dynamic memory allocation during audio processing.
Testing and Profiling
Once the system is implemented, rigorous testing and profiling are essential. Use profiling tools (e.g., Valgrind, perf, or Intel VTune) to identify bottlenecks in your multi-threaded system. Focus on minimizing processing time in the audio thread and ensuring efficient utilization of worker threads.
In conclusion, high-performance multi-threaded audio processing requires a careful balance of real-time constraints, efficient task distribution, and lock-free synchronization. Implementing delay compensation is also essential to ensure all audio signals arrive at the output in sync, preserving audio quality and coherence.